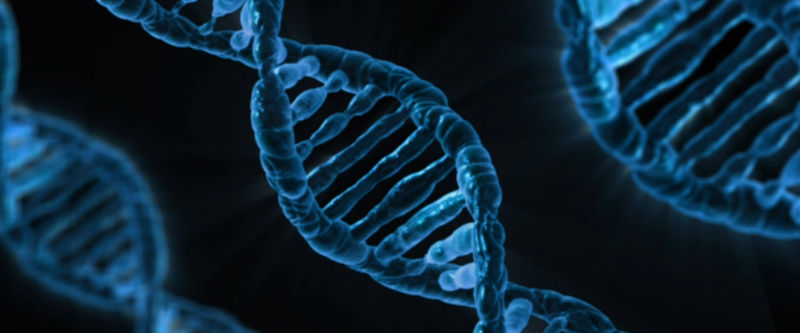
PHP Traits
What are PHP traits?
In genetics, traits refer to a characteristic or an attribute in your DNA. For instance, you have a trait for eye colour, or hair type.
In PHP traits refer to a set of common functions which can be used by multiple classes. I think of it almost as “includes for classes” (don’t quote me on that, its just a conceptual way of looking at it).
As with most programming languages, PHP is easiest learned by example, so lets delve into a PHP trait example.
Example
Assume we have two classes. One is a car class, class.Car.php, and the other is a toy class, class.Toy.php.
These classes look like this:
<?php class Car { function getModel() { return "jeep"; } } ?>
<?php class Toy { function getName() { return "doll house"; } }
We can use our new classes to print out the car’s model and the toy’s name like this:
<?php include_once(dirname(__FILE__)."/class.Toy.php"); include_once(dirname(__FILE__)."/class.Car.php"); $oToy = new Toy(); print "Toy name: ".$oToy->getName()."<p>"; $oCar = new Car(); print "Car model: ".$oCar->getModel()."<p>"; ?>
We now have two classes with methods getName and getModel. But what about if we wanted to add a method called getColour() to each class? And what if the getColour() methods worked identically, for instance, maybe we have a set number of colours to choose from in our factory, etc?
We could write the getColour() method into both classes (copy and paste). Or we could set up some base class and have a very weird inheritance (what do toys and cars really have in common???).
Another option is to use traits.
Adding PHP traits to our classes
A trait is very similar to a class. The difference is that we define it as a trait, not as a class, eg:
trait MyTrait { // methods here.. }
We then have to tell our class to use the trait through the use keyword:
class MyClass { use MyTrait; // methods.. }
We can now call the trait’s method on our class objects as if they are a part of the class. Our car / toy example above now looks like this:
PHP Trait example
class.Car.php
<?php include_once(dirname(__FILE__)."/trait.Colour.php"); class Car { use Colour; function getModel() { return "jeep"; } } ?>
Our class.Toy.php class:
<?php include_once(dirname(__FILE__)."/trait.Colour.php"); class Toy { use Colour; function getName() { return "doll house"; } } ?>
Notice how both of these classes include the trait.Colour.php file and in the class body we use use Colour; to tell it to use or import that trait’s functionality.
This is what our trait looks like:
<?php trait Colour { function getColour() { return "red"; } } ?>
And finally, to wrap it all up, our index file can now call the getColour() method on each of the two classes like this:
<?php include_once(dirname(__FILE__)."/class.Toy.php"); include_once(dirname(__FILE__)."/class.Car.php"); $oToy = new Toy(); print "Toy name: ".$oToy->getName()."<p>"; print "Toy colour: ".$oToy->getColour()."<p>"; $oCar = new Car(); print "Car model: ".$oCar->getModel()."<p>"; print "Car colour: ".$oToy->getColour()."<p>"; ?>
As you can see we can now call getColour on our classes. This allows us to stick to the DRY principle as well as not not have unnatural inheritance hierarchies.
As always, use the comments section if you have questions or comments about PHP traits.
John, a seasoned Freelance Full Stack Developer based in South Africa, specialises in delivering bespoke solutions tailored to your needs. With expertise in PHP, Laravel, Vue3, and Nuxt3, I am equipped to tackle any project, ensuring robust, scalable, and cutting-edge outcomes.
My comprehensive skill set enables me to provide exceptional freelance services both remotely and in person. Whether you’re seeking to develop an innovative application or require meticulous refinement of existing systems, I am dedicated to elevating your digital presence through unparalleled technical prowess and strategic development methodologies. Let’s connect to transform your vision into reality.